Start a User Session
Make sure you take a look at the Web Guide in order to learn the steps that need to be taken to set up Human API Connect
New user
You need to start a session for your new user before launching Connect.
Sessions guarantee the continuity of your user's experience. For instance, in the event of an abnormal termination like the browser crashing, the user can come back and resume where they left off.
Only you can start a session for your users. That's to protect you and your users from malicious "man-in-the-middle" attacks.
To start a session, issue a request to our authentication service with your client_id
, a client_user_id
and client_user_email
. The client_user_id
is the user identifier, which is any valid UTF-8 string of your choice.
Let's take a look at example code that helps us create the new session request:
// This example contains a client secret in the server context.
// DO NOT use your client secret in the browser!
// This example uses an open source request library to create requests
// You can replace it with your favorite request library
// Make sure to use the latest node version and [email protected] for this sample script to work
const fetch = require("node-fetch")
// Human API's session authentication endpoint
const authUrl = "https://auth.humanapi.co/v1/connect/token"
// Payload you send to Human API's session authentication endpoint
const requestBody = {
client_id: "--YOUR_CLIENT_ID--",
client_user_id: "--YOUR_UNIQUE_IDENTIFIER--",
client_user_email: "[email protected]",
client_secret: "--YOUR_CLIENT_SECRET--",
type: "session"
};
// Issue a POST call to Human API's authentication service
fetch(authUrl,{
method: "POST",
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(requestBody)
})
.then(response => response.json())
.then(sessionTokenData => {
console.log(sessionTokenData);
})
.catch((error) => {
console.error('Error:', error);
});
Do not ever send your client secret through the browser
Retrieving user data should not be done through the browser, it should be done via a server. Do not expose your users data by loading your client secret onto a web page.
A successful response to the request above will look like this:
{
"session_token": "--SESSION_TOKEN--",
"human_id": "70a1fd8fb4935296647f340dddf7dad6",
"expires_in": 3600 // seconds
}
The session_token
is an opaque string that identifies a the user session. You should keep it and use it to launch Connect later within the expires_in
time limit. The response also includes the human_id
, which is Human API's unique user identifier.
humanId or human_id?
humanId
andhuman_id
refer to the same concept: they are unique user identifiers provisioned by Human API.humanId
may appear in the API responses when requesting for health data;human_id
appears in the Connect user authentication process, and adheres to OAuth2 conventions.
Returning user
After the session_token
has already expired, an id_token
must be created and used for the returning user if that user connected at least one data source. The id_token
is virtually the same as the session_token in that it helps identify the user. If you try to request a new session_token
after a user connected a source, our authentication service will respond with a HTTP 403 error.
To create a request for the id_token
, the request body is slightly altered as follows:
const requestBody = {
client_id: "--YOUR_CLIENT_ID--",
client_user_id: "--YOUR_UNIQUE_IDENTIFIER--",
client_secret: "--YOUR_CLIENT_SECRET--",
type: "id" // note that this is changed from "session" to "id"
};
A successful response to the request above will look like this:
{
"token_type": "Bearer",
"id_token": "ee08580dcb5ea51554b3aced5574554c",
"id_refresh_token": "idrt-bosKV1aM8JMfsrlyce59P3StTWcLzaCP7fbG3FEMHVM"
"id_token_expires_in": 86400 // seconds
}
You should keep the id_token
and use it to launch Connect later within the id_token_expires_in
time limit.
What's the difference between session_token and id_token?
Even though
session_token
andid_token
serve virtually the same purpose in identifying the user, we useid_token
as a more secure method of authorizing a user after they have already connected a data source. Additionally,session_tokens
expire in 1 hr (3600 seconds), whileid_tokens
expire in 1 day (86400 seconds).
What should I do with the id_refresh_token?
While the token is not used actively today, please save the token in your database.
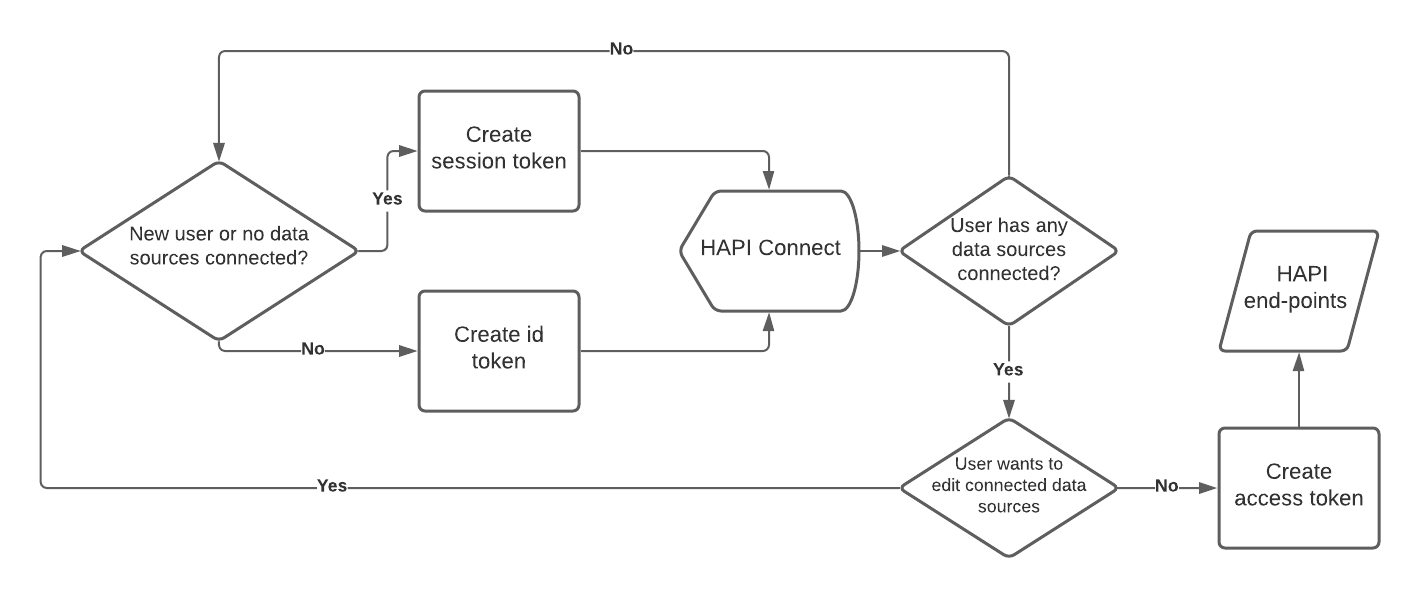
Authorization token workflow
Common errors
Here is a list of the common errors that can be encountered while trying to start or retrieve a session:
errorCode | Description | How to Fix |
---|---|---|
INVALID_TOKEN_TYPE | The specified token type parameter is invalid | Make sure you are sending type: "session" or type: "id" |
ID_TOKEN_GENERATION_NOT_ALLOWED | User is not allowed to generate ID tokens | Reach out to us at [email protected] |
CLIENT_TOKEN_GENERATION_NOT_ALLOWED | client_id is not allowed to generate client tokens | Reach out to us at [email protected] |
INVALID_CLIENT_ID | Invalid client_id | Make sure that you are sending the correct client_id |
INVALID_CLIENT_ID_OR_SECRET | Invalid client_id or client_secret | Make sure that you are sending the correct client_id and client_secret |
SESSION_TOKEN_GENERATION_NOT_ALLOWED | Session token time window expired: no longer allowed to generate session tokens | If the user had already connected a source, request for an id_token. If this is not hte issue, reach out to us at [email protected] |
UNKNOWN_CLIENT_USER_ID | Unknown client_user_id | When requesting type: "id" , make sure that you have generated a session token for the user and that they have connected sources. |
NOT_AUTHORIZED | Not authorized to perform this action | Reach out to us at [email protected] |
Updated about 3 years ago