Android Guide
How to use Human API to get health data easily from your mobile app
This page will help you get started with Human API for Android. You'll be up and running in no time! If you haven't already, please create a developer account as described on the Start Here page and come back here when you're done.
Overview
As described in Start Here, there are two main parts to integrating Human API with your application:
- A: Authentication: Allowing your users to authenticate their data
- B: Data Retrieval: Accessing authenticated user data
This guide will walk through the implementation of the Human API Android SDK to handle part A by allowing your users to authenticate their data within your application via the Human Connect popup . After the authentication process is complete, you will have the credentials needed to access user data from your server to complete part B as detailed in the Data Retrieval section of the documentation.
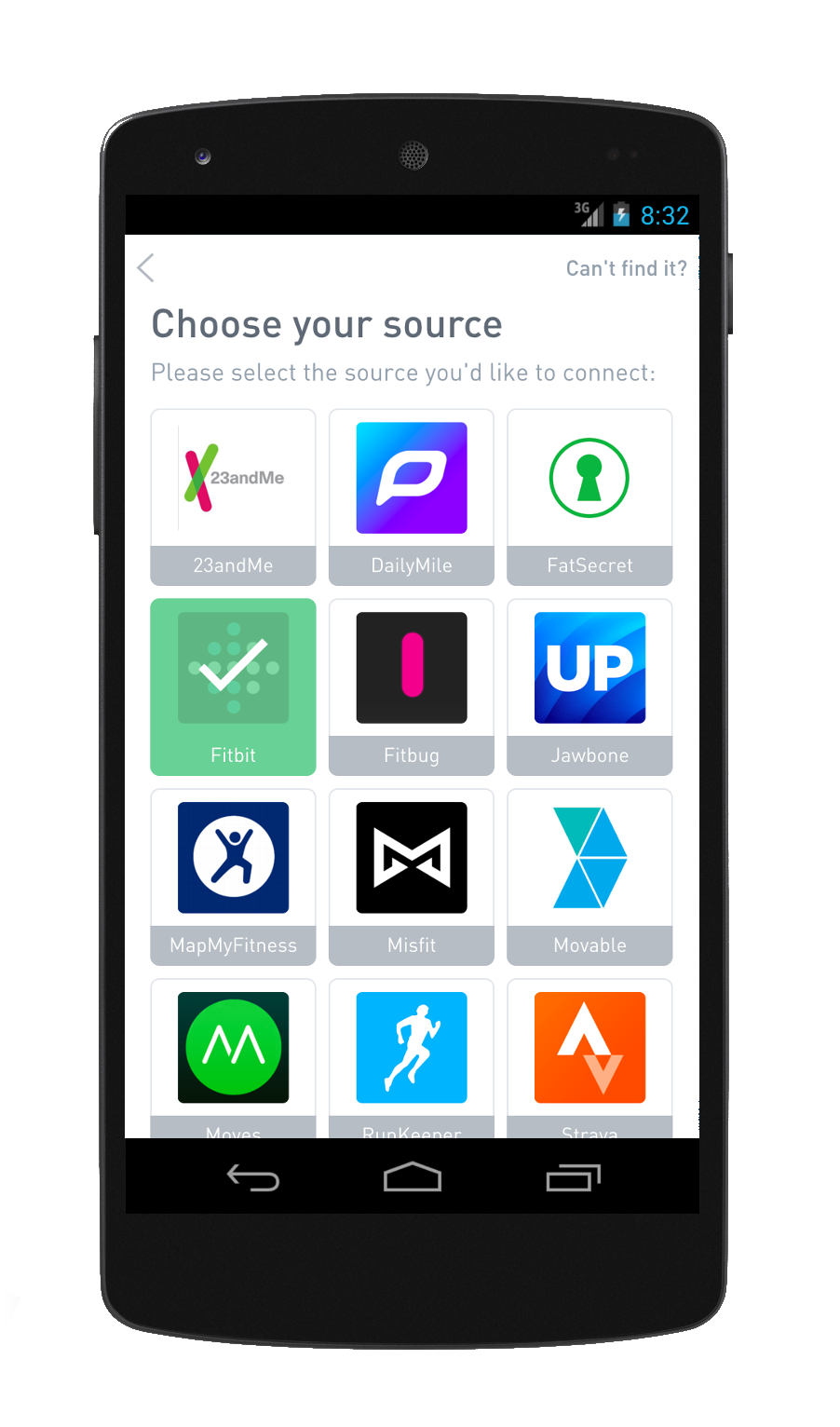
Connect on Android
Add the SDK to your Application
The HumanAPI Android SDK consists of one package co.humanapi.connectsdk
, which is browser-based UI to handle the launch and callback functions of Human Connect.
Import SDK module for Android Studio
- Clone the SDK with
$ git clone https://github.com/humanapi/humanapi-android-client
- Copy the
humanapi-sdk
folder into your project's "app" folder - Import
humanapi-sdk
as a module for your application - Go to File -> New -> Import Module -> Source Directory -> Browse the project path for
humanapi-sdk
- Specify the Module Name as
:humanapi-sdk
in the dialog box - Let Android Studio build the project.
- Open
build.gradle (Module:app)
file and add the following line in the dependencies block:
compile project(':humanapi-sdk')
- Press the “sync now” link to start a sync of gradle files
Add Android Permissions
If you don't have it already, be sure to add android.permission.INTERNET
to your application's AndroidManifest.xml
so that the SDK can access the internet.
<!--Add to app AndroidManifest.xml file within the <manifest> but outside <application> tags-->
<uses-permission android:name="android.permission.INTERNET" />
Configure and Launch Human Connect
Now that you have installed the SDK, it's time to set up the authentication flow.
A user authenticates Human API by clicking the Connect Health Data button and authenticating their data directly with your application via Human Connect.

You can easily embed this authentication process directly into your Android application.
First, add the button above (or another button of your choosing) to the Activity you would like to launch Connect from. Next, connect this button to a function (we'll use onConnect()
) to launch Human Connect in three steps:
1. Create an Intent for Human Connect
Use the code below to create an Intent for Human Connect targeting the ConnectActivity class in the SDK:
Intent intent = new Intent(this, co.humanapi.connectsdk.ConnectActivity.class);
2. Add Configuration Options to the Intent
Bundle b = new Bundle();
b.putString("client_id", "659e9bd58ec4ee7fa01bc6b4627cb37e5c13ec21");
b.putString("auth_url", "http://10.0.2.2:3000/sessionToken");
b.putString("client_user_id", "[email protected]");
b.putString("language", "en");
//PublicToken (mandatory for existing users)
//b.putString("public_token", "e56fa0350866bcf266da442cb974d84e");
intent.putExtras(b);
Human Connect needs to be passed a few values for it to load correctly. These values are:
Property | Description |
---|---|
client_id | Unique ID of your developer portal application (found on the application settings page). |
auth_url | Custom endpoint on your server to handle the user token exchange. (You can add the default for now, we'll be implementing this in the second half of this guide.) |
client_user_id | Unique ID of the user in your system. This will be returned in the final step so that you can associate Human API credentials with the correct user. |
language (optional) | Localization option. See all possilbe values here. |
public_token | Token used when launching Connect for existing users. You'll get this token after the first successful authentication, so it's okay to leave it out for now. |
PublicToken
You will receive a
publicToken
at the end of the user authentication process. This value must be passed into Human Connect every time the user opens the popup thereafter. It is not necessary for the first launch.
3. Launch the Activity for Human Connect
Finally, use startActivityForResult
to launch the Activity for Human Connect with the Intent and Bundle created above:
startActivityForResult(intent, HUMANAPI_AUTH);
Complete Launch Implementation
Altogether, your launch code for Human Connect should now look something like this:
static final int HUMANAPI_AUTH = 1;
public void onConnect(View view) {
/* 1. Create an Intent for Human Connect */
Intent intent = new Intent(this, co.humanapi.connectsdk.ConnectActivity.class);
/* 2. Add Bundle with configuration options */
Bundle b = new Bundle();
b.putString("client_id", "659e9bd58ec4ee7fa01bc6b4627cb37e5c13ec21");
b.putString("auth_url", "http://10.0.2.2:3000/sessionToken");
b.putString("client_user_id", "[email protected]");
b.putString("language", "en");
//PublicToken (mandatory for existing users)
//b.putString("public_token", "e56fa0350866bcf266da442cb974d84e");
intent.putExtras(b);
/* 3. Launch Human Connect Activity */
startActivityForResult(intent, 2);
}
That is all that is needed to correctly launch Human Connect to allow your users to authenticate their data. The second half of the authentication process will occur on your server at the auth_url
endpoint.
[Server-Side] Handle Token Exchange
When a user authenticates a new data source in Human Connect, the Connect SDK will POST a sessionTokenObject
to your server for final processing via the auth_url
specified on launch.
To complete the token exchange process, you should:
-
Add your
clientSecret
to thesessionTokenObject
(clientSecret can be found on the app settings page in the developer portal) -
POST the modified
sessionTokenObject
from your server to Human API Tokens Endpoint:
https://user.humanapi.co/v1/connect/tokens
-
Retrieve and store
humanId
,accessToken
andpublicToken
on your server with the user model to be used to query user data from Human API.
(See here for more information on what these values are.) -
Return status
200 OK
with payload containingpublicToken
to store on device for future launches of Human Connect:
{ publicToken: "2767d6oea95f4c3db8e8f3d0a1238302" }
Here's an example of what this endpoint might look like in Node.js:
//Endpoint for specified 'authURL'
app.post('/sessionToken', function(req, res, next) {
var sessionTokenObject = req.body;
// grab client secret from app settings page and `sign` `sessionTokenObject` with it.
sessionTokenObject.clientSecret = '<Your-Client-Secret>';
request({
method: 'POST',
uri: 'https://user.humanapi.co/v1/connect/tokens',
json: sessionTokenObject
}, function(err, resp, body) {
if(err) return res.send(422);
//Use these values to determine which user launched Connect
console.log("clientId ="+ body.clientId);
console.log("clientId ="+ body.clientId);
/* Human API credentials.
Save these with the user model in your system. */
console.log("humanId = " + body.humanId);
console.log("accessToken = "+ body.accessToken);
console.log("publicToken = "+ body.publicToken);
//Send back publicToken to Android app
var responseJSON = {publicToken: body.publicToken};
res.setHeader('Content-Type', 'application/json');
res.status(201).send(JSON.stringify(responseJSON));
});
});
For more information on the server implementation, see the detailed guide: Finalizing User Authentication.
Handle Callbacks from Human Connect
When the token exchange process is complete on your server, the Human API SDK will deliver your response to onActivityResult
under the RESULT_OK
result code.
Here, you should save the returned publicToken
on the device so that you can supply it in the Intent whenever the user launches Connect again in the future.
RESULT_CANCELED
occurs when the user closes the Connect window without authenticating a source. In this case, nothing is sent to the specified auth_url
so there aren't any tokens or user data to retrieve. Implementation of this callback is this therefore optional.
/** Called when result returned */
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode != HUMANAPI_AUTH) {
return; // incorrect code
}
if (resultCode == RESULT_OK) {
Log.d("hapi-home", "Authorization workflow completed");
Bundle b = data.getExtras();
Log.d("hapi-home", ".. public_token=" + b.getString("public_token"));
} else if (resultCode == RESULT_CANCELED) {
Log.d("hapi-home", "Authorization workflow cancelled");
}
}
That's it! Now that you have the user's humanId
and accessToken
, you can start querying their data on your server for display in your app.
See the documentation in the Data Retrieval section of the documentation for more information.
Updated over 6 years ago