iOS Guide
How to use Human API to get health data easily from your mobile app
This page will help you get started with Human API for iOS. You'll be up and running in no time! If you haven't already, please create a developer account as described on the Start Here page and come back here when you're done.
Overview
There are two main parts to integrating Human API with your application:
- A: Allowing your users to authenticate their data
- B: Accessing authenticated user data
This guide will walk through the implementation of the Human API iOS SDK to handle part A by allowing your users to authenticate their data within your application via the Human Connect popup . After the authentication process is complete, you will have the credentials needed to access user data from your server to complete part B as detailed in the Data Retrieval section of the documentation.
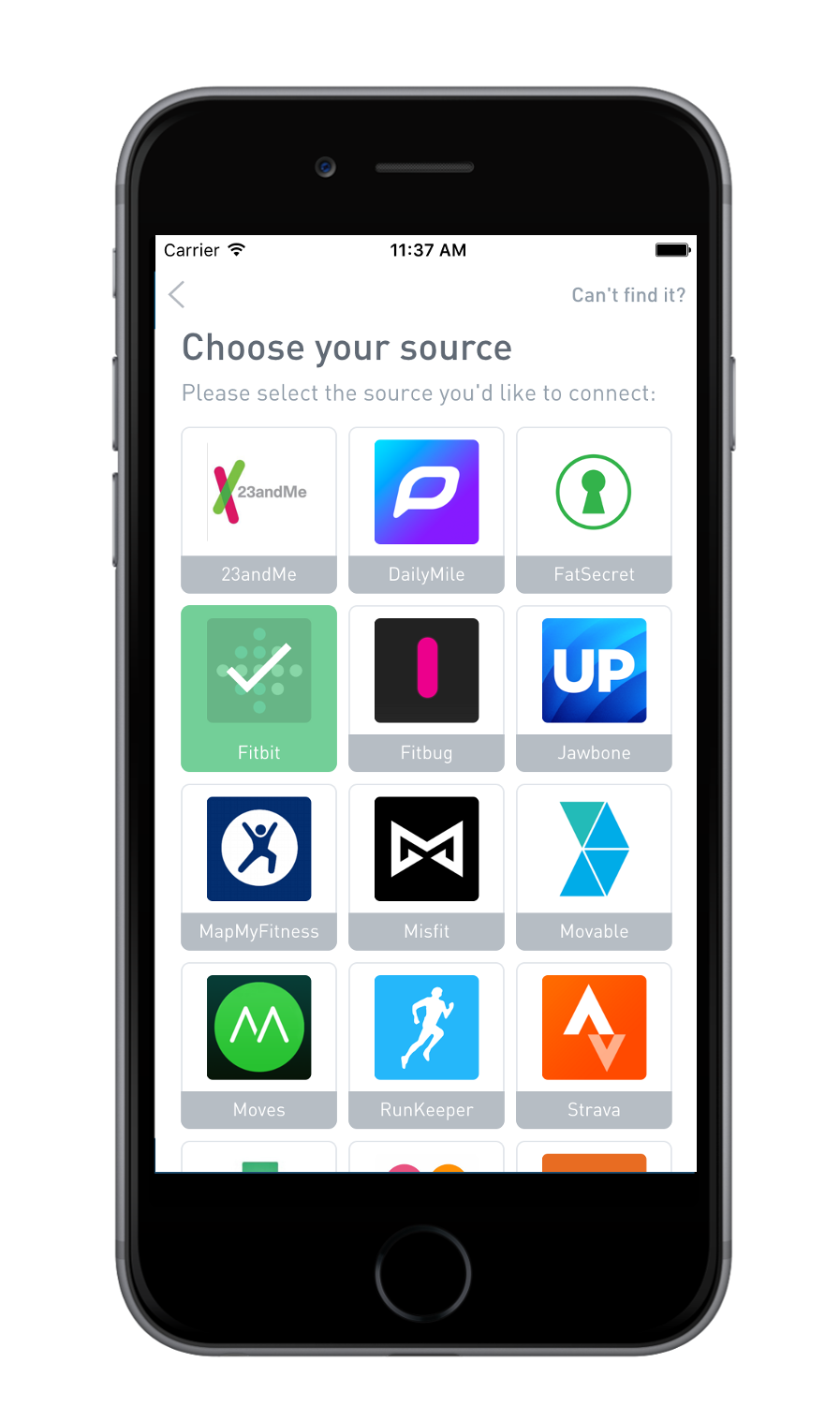
Connect on iOS
Add the SDK to your Application
Copy the HumanAPI
folder from the master branch of the iOS SDK and place it in the base level of your project directory.
Configure and Launch Human Connect
Now that you have the SDK, it's time to set up the authentication flow.
A user authenticates Human API by clicking the Connect Health Data button and authenticating their data directly with your application via Human Connect.

You can easily embed this authentication process directly into your iOS application.
First, add the button above (or another button of your choosing) as a UIButton
to the view you would like to launch Connect from and link it to an IBAction
in the view controller. You can then use this button to launch Human Connect in the following three steps:
1. Import the SDK ViewController and Protocol
In your ViewController.h file:
#import "HumanConnectViewController.h"
at the top- Add
<HumanAPINotifications>
protocol to the ViewController
e.g.@interface ViewController : UIViewController <HumanAPINotifications>
2. Instantiate the HumanConnectViewController
Within the IBAction function you created, instantiate the HumanConnectViewController
:
NSString *myClientID = @"<Your-Client-ID>";
NSString *authURL = @"http://localhost:3000/sessionToken";
HumanConnectViewController *hcvc = [[HumanConnectViewController alloc] initWithClientID:myClientID andAuthURL:authURL];
hcvc.delegate = self;
[self presentViewController:hcvc animated:YES completion:nil];
let clientId : String = "659e9bd58ec4ee7fa01bc6b4627cb37e5c13ec21"
let authURL : String = "http://localhost:3000/sessionToken"
let hcvc : HumanConnectViewController = HumanConnectViewController(clientID: clientId, andAuthURL: authURL);
hcvc.delegate = self;
self.presentViewController(hcvc, animated: true, completion: nil);
You will need to pass these two values into the constructor:
Name | Description |
---|---|
clientId | Unique ID of your developer portal application (found on the application settings page). |
authURL | Custom endpoint on your server to handle the user token exchange. (You can add the default for now, we'll be implementing this in the second half of this guide.) |
3. Launch Connect for the User
Use startConnectFlowForNewUser: (NSString *)userId
to launch Connect for a new user and pass it the unique ID of the user in your system (This will be returned in the final step so that you can associate Human API credentials with the correct user.)
For now, this is all you need, but be aware that the next time the user launches Connect, you will need to use startConnectFlowFor: (NSString *)userId andPublicToken: (NSString *)publicToken
. You will get this token after the first successful authentication.
[hcvc startConnectFlowForNewUser:locaUser.email];
//If you have a publicToken for the user, supply it to Human Connect on launch
//[hcvc startConnectFlowFor:localUser.email andPublicToken:localUser.publicToken];
hcvc.startConnectFlowForNewUser(localUser.email);
//If you have a publicToken for the user, supply it to Human Connect on launch
// hcvc.startConnectFlowFor("localUser.email", andPublicToken: "localUser.publicToken")
PublicToken
You will receive a
publicToken
at the end of the user authentication process. This value must be passed into Human Connect every time the user opens the popup thereafter. It is not necessary for the first launch.
Complete Launch Implementation
Altogether, your launch code for Human Connect should now look something like this:
/* ViewController.h */
#import <UIKit/UIKit.h>
#import "HumanConnectViewController.h"
@interface ViewController : UIViewController <HumanAPINotifications>
@end
/* ViewController.m */
#import "ViewController.h"
@interface ViewController ()
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (IBAction)launchHumanConnect:(id)sender {
NSString *myClientID = @"<Your-Client-ID>";
NSString *authURL = @"http://localhost:3000/sessionToken";
HumanConnectViewController *hcvc = [[HumanConnectViewController alloc] initWithClientID:myClientID andAuthURL:authURL];
hcvc.delegate = self;
[self presentViewController:hcvc animated:YES completion:nil];
[hcvc startConnectFlowForNewUser:locaUser.email];
//If you have a publicToken for the user, supply it to Human Connect on launch
//[hcvc startConnectFlowFor: localUser.email
// andPublicToken: localUser.publicToken];
}
@end
import UIKit
class ViewController: UIViewController, HumanAPINotifications {
override func viewDidLoad() {
super.viewDidLoad()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
@IBAction func launchConnect(sender: UIButton) {
let clientId : String = "<Your-Client-ID>"
let authURL : String = "http://localhost:3000/sessionToken"
let hcvc : HumanConnectViewController = HumanConnectViewController(clientID: clientId, andAuthURL: authURL);
hcvc.delegate = self;
self.presentViewController(hcvc, animated: true, completion: nil);
//Use your local user identifier here
hcvc.startConnectFlowForNewUser("localUser.email");
//If you have a publicToken for the user, supply it to Human Connect on launch
// hcvc.startConnectFlowFor("localUser.email", andPublicToken: "localUser.publicToken")
}
}
iOS 9+
If your authURL does not have SSL (https) such as the localhost example above, you will need to configure an App Transport Security Exception so that iOS does not block the POST request to an insecure URL. See the guide here for more information.
That is all that is needed to correctly launch Human Connect to allow your users to authenticate their data. The second half of the authentication process will occur on your server at the authURL
endpoint.
[Server-Side] Handle Token Exchange
When a user authenticates a new data source in Human Connect, the Connect SDK will POST a sessionTokenObject
to your server for final processing via the authURL
specified on launch.
To complete the token exchange process, you should:
-
Add your
clientSecret
to thesessionTokenObject
(clientSecret can be found on the app settings page in the developer portal) -
POST the modified
sessionTokenObject
from your server to Human API Tokens Endpoint:
https://user.humanapi.co/v1/connect/tokens
-
Retrieve and store
humanId
,accessToken
andpublicToken
on your server with the user model to be used to query user data from Human API.
(See here for more information on what these values are.) -
Return status
200
or201
with payload containingpublicToken
to store on device for future launches of Human Connect:
{ publicToken: "2767d6oea95f4c3db8e8f3d0a1238302" }
Here's an example of what this endpoint might look like in Node.js:
//Endpoint for specified 'authURL'
app.post('/sessionToken', function(req, res, next) {
var sessionTokenObject = req.body;
// grab client secret from app settings page and `sign` `sessionTokenObject` with it.
sessionTokenObject.clientSecret = '<Your-Client-Secret>';
request({
method: 'POST',
uri: 'https://user.humanapi.co/v1/connect/tokens',
json: sessionTokenObject
}, function(err, resp, body) {
if(err) return res.send(422);
//Use these values to determine which user launched Connect
console.log("clientId ="+ body.clientId);
console.log("clientId ="+ body.clientId);
/* Human API credentials.
Save these with the user model in your system. */
console.log("humanId = " + body.humanId);
console.log("accessToken = "+ body.accessToken);
console.log("publicToken = "+ body.publicToken);
//Send back publicToken to Android app
var responseJSON = {publicToken: body.publicToken};
res.setHeader('Content-Type', 'application/json');
res.status(201).send(JSON.stringify(responseJSON));
});
});
For more information on the server implementation, see Finalizing User Authentication.
Handle Callbacks from Human Connect
When the token exchange process is complete on your server, the Human API SDK will deliver your response to the (void) onConnectSuccess: (NSDictionary *)data
& (void) onConnectFailure:(NSString *)error
callbacks on the user's device.
onConnectSuccess
Here, you should save the returned publicToken
on the device so that you can supply it to the HumanConnectViewController whenever the user launches Connect again in the future.
onConnectFailure
This is called when there is an error from Connect. Implementation of this callback is this therefore optional.
/** Connect success handler */
- (void)onConnectSuccess:(NSDictionary *)data
{
NSLog(@"Connect success! publicToken=\n %@", data);
//Notify user of success
//Save publicToken with local user for subsequent Human Connect launches
}
/** Connect failure handler */
- (void)onConnectFailure:(NSString *)error
{
NSLog(@"Connect failure: %@", error);
}
That's it! Now that you have the user's humanId
and accessToken
, you can start querying their data on your server for display in your app.
See the documentation in the Data Retrieval section of the documentation for more information.
Updated over 6 years ago